3 JavaScript Document getElementsByClassName Projects with the help of ChatGPT
So it’s been a week since I’ve restarted my bootcoding journey. I had to stop my boot camp in May 2023 due to a full-time work schedule and an inability to handle everything. It’s been over a year and I’m so happy to be back.
I’m also excited to see the different ways I can use ChapGPT to assist me in my learning.
This brings us to the topic of this post…How I’m using ChatGPT to help me practice JavaScript Document getElementsByClassName and other HTML DOM properties.
Table of Contents
ChatGPT and Coding
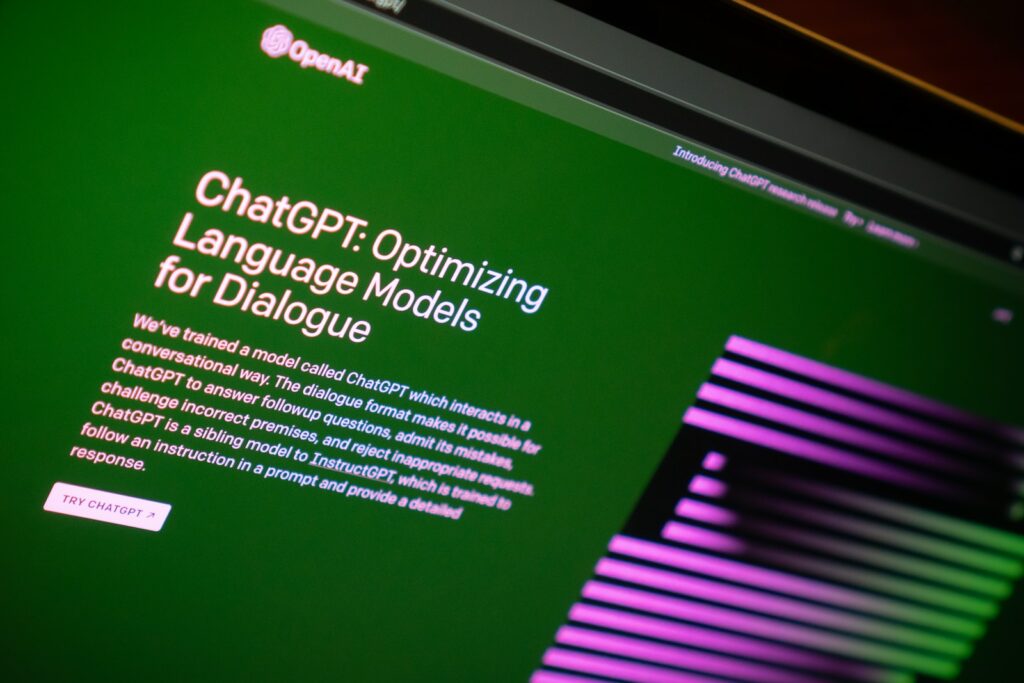
I’m sure you’ve heard of ChatGPT and its impact on the world, especially technology and coding. Many are saying ChatGPT and Artificial Intelligence (AI) will take over the jobs of software developers and the like.
And they’re right it will…eventually. But as of right now just knowing how to write code doesn’t do anyone any good if we don’t know how to apply it.
As you’ll see this is the beauty of ChatGPT for coding. This is especially for new coders, who are not only learning how to write code but also learning problem-solving skills and how to apply what they’re learning.
Using ChatGPT to Help Practice Coding
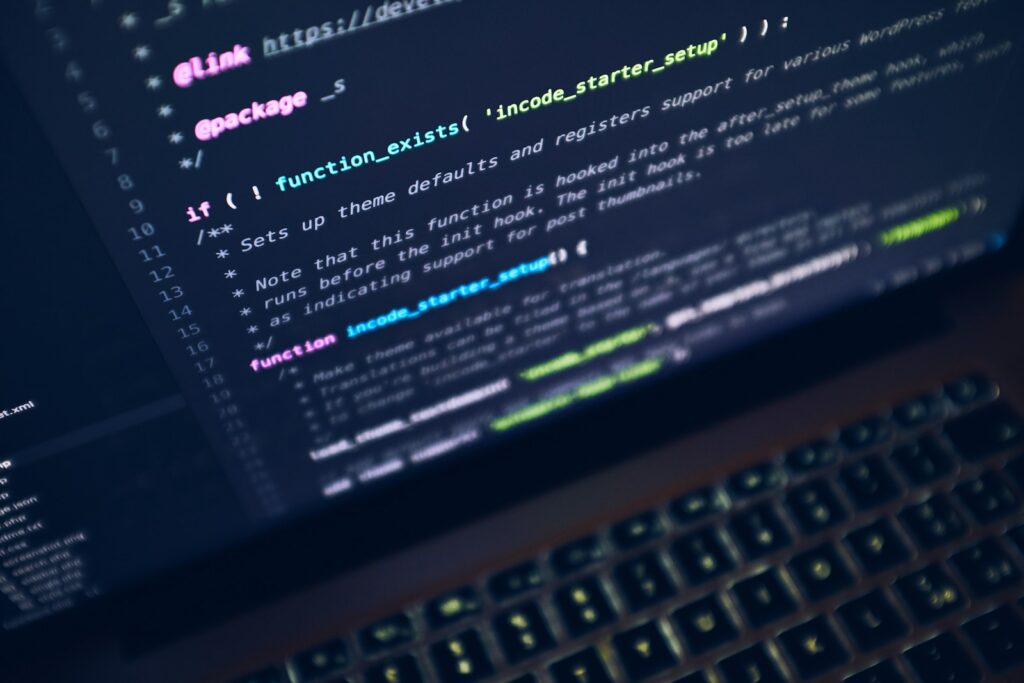
One thing to know about me is that I am a hands-on learner. I learn by jumping in and getting my hands dirty.
While there is a lot to learn in my coding boot camp, I don’t do well with abstract theories and exercises. A lot of the examples are given in ways that have no real-life application. This is why I decided to use my experience with WordPress to help me actually apply what I’m learning.
However, you don’t know what you don’t know and although I’m learning what the JavaScript getElementsByClassName method is, I still don’t know what it does and how it works in the real world.
If you’d like to learn more about HTML DOM Documents and JavaScript, check out W3schools.
This is where ChatGPT comes into play.
I was in a workshop learning how to use ChatGPT as a personal assistant. The presenter did an amazing job and I got really excited about the possibilities.
Since I’m just learning all these great JavaScript concepts and methods, I’d wanted to way to practice them in real-life situations.
So, I asked ChatGPT the following question:
“I’m attending a coding boot camp to learn to become a front-end web developer. Can you give me some simple projects to practice using getElementsByClassName, getElementById, and getElementByTagName, include the HTML”
This idea came to me as I was watching videos and working through some of my classes. I understood what was being taught, but couldn’t figure out HOW to practice it.
I thought it would be cool to have some specific projects or tasks related to what I was learning.
This is what ChatGPT gave me:
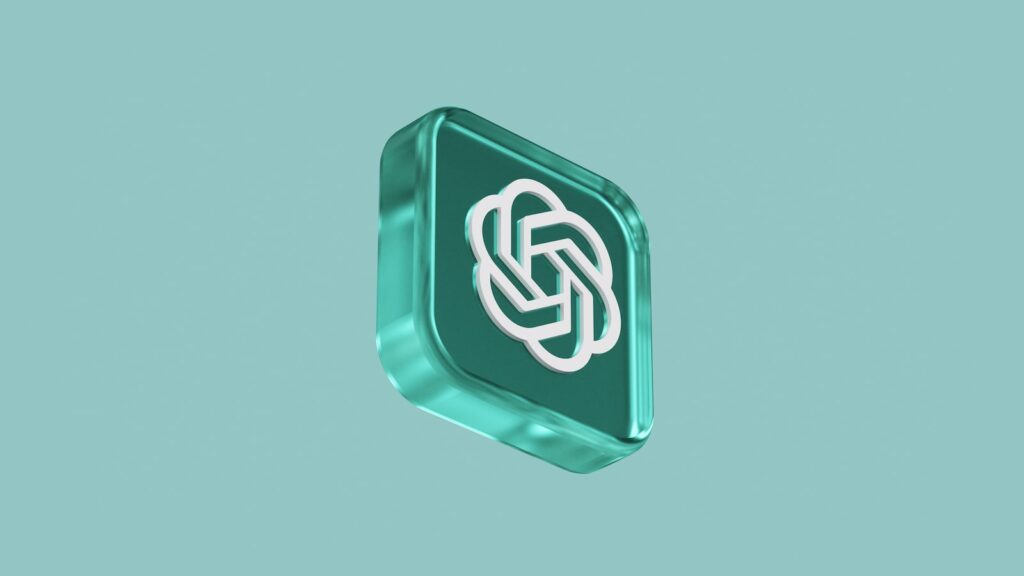
Certainly! Here are three simple projects for practicing the use of getElementsByClassName
, getElementById
, and getElementsByTagName
in JavaScript. I’ll provide you with the HTML and JavaScript code for each project.
Project 1: Using JavaScript Document getElementsByClassName
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Project 1 - getElementsByClassName</title>
</head>
<body>
<div class="box red">Red Box</div>
<div class="box green">Green Box</div>
<div class="box blue">Blue Box</div>
<button id="changeColorBtn">Change Colors</button>
</body>
</html>
JavaScript:
// Function to change the color of elements with the 'box' class
function changeColors() {
var boxes = document.getElementsByClassName('box');
for (var i = 0; i < boxes.length; i++) {
boxes[i].style.backgroundColor = getRandomColor();
}
}
// Function to generate a random color
function getRandomColor() {
var letters = '0123456789ABCDEF';
var color = '#';
for (var i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
document.getElementById('changeColorBtn').addEventListener('click', changeColors);
When you click the “Change Colors” button, this code will change the background color of all elements with the class “box” to a random color.
Project 2: Using getElementById
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Project 2 - getElementById</title>
</head>
<body>
<h1 id="headline">This is a headline</h1>
<button id="changeTextBtn">Change Headline</button>
</body>
</html>
JavaScript:
// Function to change the text of the element with the id 'headline'
function changeHeadline() {
var headline = document.getElementById('headline');
headline.innerText = 'New Headline Text';
}
document.getElementById('changeTextBtn').addEventListener('click', changeHeadline);
When you click the “Change Headline” button, this code will change the text of the element with the id “headline” to “New Headline Text.”
Project 3: Using getElementsByTagName
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Project 3 - getElementsByTagName</title>
</head>
<body>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<button id="addItemBtn">Add Item</button>
</body>
</html>
JavaScript:
// Function to add a new item to the list
function addItem() {
var ul = document.getElementsByTagName('ul')[0];
var li = document.createElement('li');
li.innerText = 'New Item';
ul.appendChild(li);
}
document.getElementById('addItemBtn').addEventListener('click', addItem);
When you click the “Add Item” button, this code will add a new item to the unordered list.
These simple projects should help you practice using getElementsByClassName
, getElementById
, and getElementsByTagName
in JavaScript.
Application
Once I received these practice projects I got straight to work!
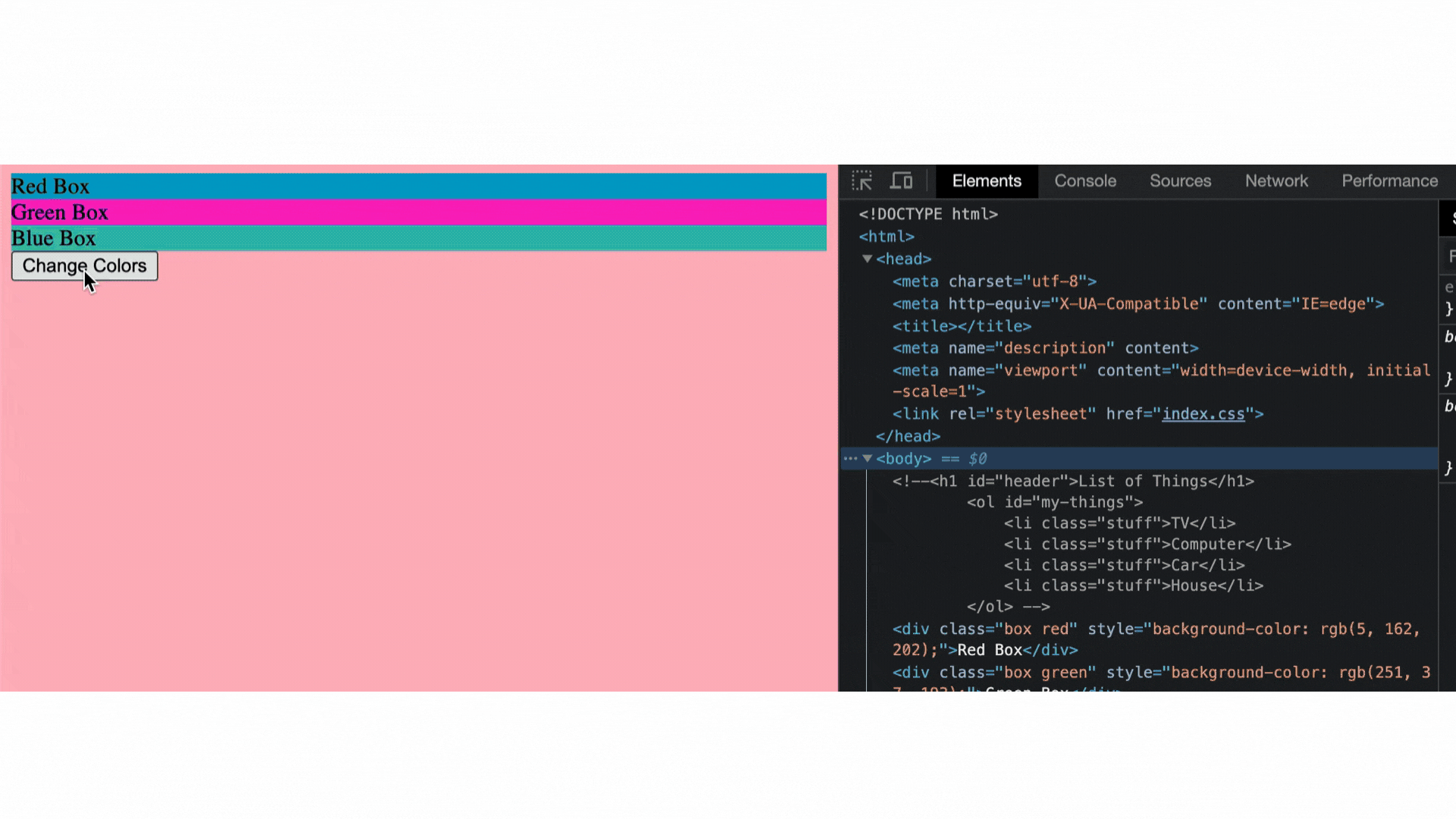