My Online JavaScript Bootcamp Journey | Pt 1 | Conquering My 1st Mid-Level Assessment
Enrolling in an online JavaScript Bootcamp is a significant step in my coding journey. My 1st mid-level assessment was an intimidating hurdle, but with dedication and hard work, I managed to overcome my doubts.
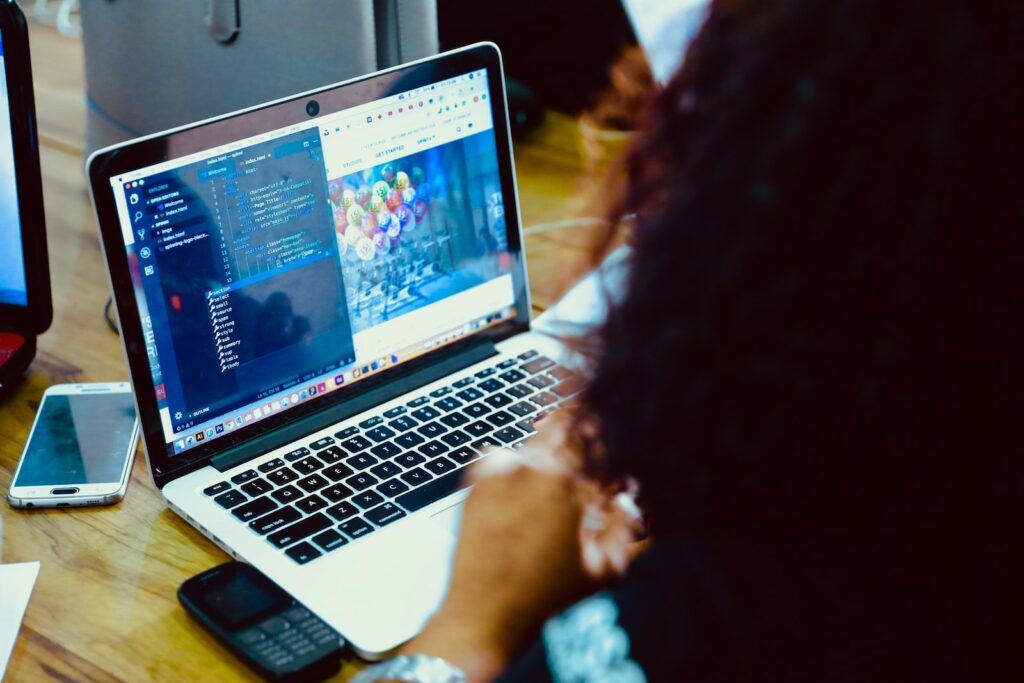
In this blog post, I’ll share this part of my journey and the key steps that led to my success, including writing a constructor function, instigating new books, and even a live coding assessment where I used the push method to add a new name to a list of other names in an array.
Navigating My 1st Mid-Level Assessment in My Online JavaScript Bootcamp
Table of Contents
Preparing for the Online JavaScript Bootcamp Assessment:
Before the assessment, I dedicated myself to reviewing the material covered in the bootcamp. This included revisiting key concepts and practicing coding exercises related to constructor functions, object instantiation, and array manipulation.
While going through the beginning of level 1 I also learned a lot about JavaScript HTML DOM methods like:
- document.getElementsById
- document.getElementsByClassName
- document.getElementsByTagName
Understanding Constructor Functions
A constructor function is a fundamental concept in JavaScript that allows you to create objects based on a blueprint. In preparation, I delved deep into the nuances of constructor functions, understanding how they work and how to use them effectively.
Homework Assignment
The homework assignment that prepared us for the assessment involved writing a constructor function and instigating new books. This task tested our understanding of object-oriented programming in JavaScript. I took this as an opportunity to put theory into practice.
Writing the Constructor Function
To tackle the homework assignment, I created a constructor function for books. This function defined the properties and methods that each book object would have. I made sure to be precise in my code, paying attention to details.
//Constructor Function for the Books
function Book(title, author, year) {
this.title = title;
this.author = author;
this.year = year;
//this.getDetails = function () {
//console.log("Title: " + this.title + ", " + "Author: " + this.author + ", " + "Year: " + this.year);
//}
}
//Write constructor function with method to instanitate new books
Book.prototype.getDetails = function () {
console.log("Title: " + this.title + ", " + "Author: " + this.author + ", " + "Year: " + this.year);
}
Instigating New Books
Creating instances of objects using the constructor function was the next step. I instantiated several books, each with different properties. This step required careful attention to syntax and the correct use of the ‘new’ keyword.

Testing and Debugging
As with any coding task, testing and debugging were crucial. I ran various test cases to ensure that my constructor function and the instantiation of books worked as intended. Debugging was a significant part of the process, and I learned a lot from resolving issues.
Live Coding Assessment
In addition to the homework assignment, I had to perform a live coding assessment. During this task, I was only given the information and the expected outcome.
//Add Robert to the end of the list using the push Array Methods
const names = ["Jerry", "Adam"]
const person = { firstName: "Robert", lastName: "Jones", age: 37 }
//Expected Output: [ "Jerry", "Adam", "Robert"]
I had to determine what actually needed to be done and what tool I needed to get the expected outcome. I decided to use the Array push method to add a new name to a list of other names in an array. This live assessment challenged my ability to think on my feet and apply array manipulation skills under time pressure.
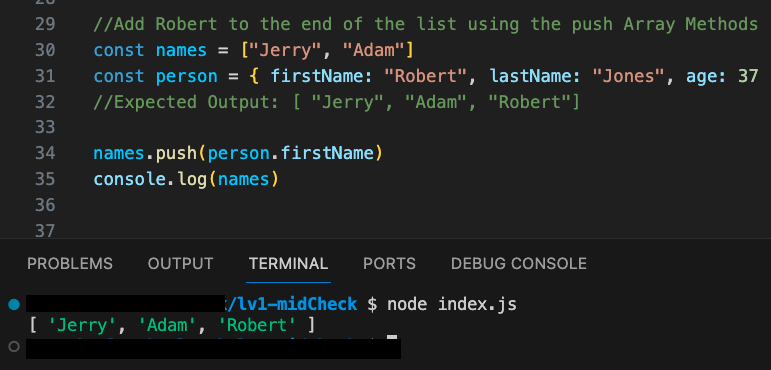
Seeking Help and Collaboration: A Pillar of Online JavaScript Bootcamps
Throughout the preparation, I didn’t hesitate to seek help from instructors, peers, and online resources, a practice encouraged in online JavaScript Bootcamps. Collaborating with others allowed me to gain different perspectives and insights, making me more confident in my knowledge.
Staying Calm and Confident
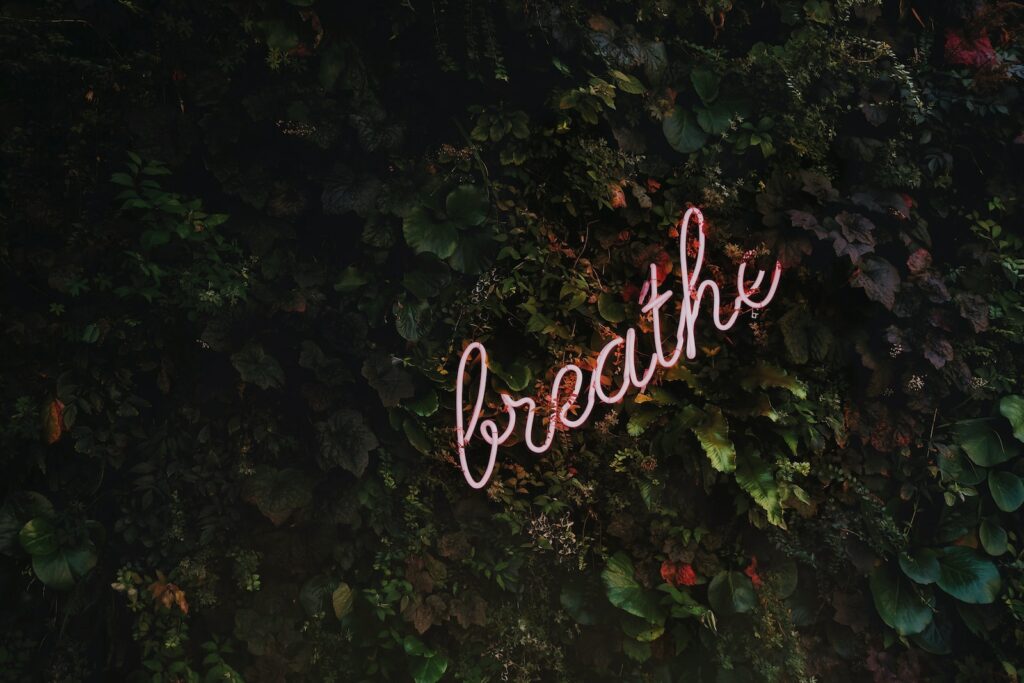
On the day of the assessment, I reminded myself to stay calm and trust the process, a mindset often discussed in online JavaScript Bootcamps. Nervousness is natural, but my preparation and practice gave me the confidence to tackle the challenge.
Celebrating Success
Passing the mid-level assessment in the online JavaScript Bootcamp was a significant achievement. It proved that my dedication and hard work had paid off. I celebrated this success with a sense of accomplishment and pride in my newfound coding skills, a hallmark of online JavaScript Bootcamp success.
Conclusion: Thriving in the Mid-Level Challenges of an Online JavaScript Bootcamp
Taking and passing the mid-level assessment in an online JavaScript Bootcamp was a transformative experience. It pushed me to learn and apply essential coding concepts, such as constructor functions, object instantiation, and array manipulation. The journey is challenging but ultimately rewarding, demonstrating the power of dedication and persistence in the world of coding and the invaluable experience of an online JavaScript Bootcamp.