10 Exercises I’m Using to Master Addition JavaScript Operators
Hey there, fellow code explorers! 👋 As I dive deeper into the world of JavaScript, I’ve encountered a fascinating duo of JavaScript Operators that I couldn’t wait to share with you.
Have you ever wondered about the difference between +=
and +
in JavaScript?
It’s one of those coding curiosities that often pops up when you’re just starting your journey as a new coder. Don’t worry; I’m here learning with you!
In this blog post, I’ll break down what I learned in the most techy-belle-friendly way I can, so we can unravel the mystery of these operators together. So, grab your coding cape, and let’s embark on this adventure into the realm of Addition Assignment (+=)
and Addition (+)
JavaScript Operators. 🚀💻✨
What are JavaScript Addition Operators?
JavaScript Addition Operators refer to two specific operators: +=
and +
. The +=
operator is known as the Addition Assignment operator, while the +
operator is simply the Addition operator.
In JavaScript, the += operator combines addition and assignment functionalities. It allows you to increment the value of a variable by adding a specific number to its existing value.
For example, if you have a variable x with the value of 5, using the += operator, you can increase the value of x by 3, resulting in x becoming 8.
On the other hand, the + operator in JavaScript is used to perform addition between two values. When used with two numbers, it adds them together and returns the sum. Additionally, it can also concatenate strings.
For instance, if you have the strings “Hello” and “World” and you use the + operator to combine them, you would get the result “HelloWorld”.
Understanding the difference between these two operators is crucial for writing efficient and concise code. It allows you to perform mathematical calculations and string manipulations in JavaScript effectively. So, let’s dive deeper into these operators and explore their practical applications. 🚀💡💻
In Simpler Terms…
In JavaScript, the Addition Assignment (+=)
and Addition (+
) are JavaScript Operators used for different purposes.
+=
(Addition Assignment Operator):
+=
is a compound assignment operator.- It is used to add the value on the operator’s right-hand side to the value on the left-hand side and then assign the result back to the left-hand side variable.
- It is commonly used to update the value of a variable by adding another value to it.
- Example:
x += 5;
is equivalent tox = x + 5;
It adds 5 to the current value ofx
and stores the result back inx
.
2. +
(Addition Operator):
+
is a binary operator for addition.- It is used to add two values together or concatenate strings when used with strings.
- When used with numbers, it performs addition.
- When used with strings, it concatenates (joins) the strings.
- Example with numbers:
3 + 5
results in 8. - Example with strings:
"Hello" + " " + "World"
results in the string “Hello World”.
Here are 10+ simple exercises to practice the difference between these two JavaScript Operators:
let x = 5;
let y = 3;
// Using +=
x += 2; // This updates the value of x to 7 (5 + 2)
// Using +
let z = x + y; // This calculates the sum of x and y, so z is 10 (7 + 3)
In order to really drive this home, I like to open up my Visual Studio Code, type out the examples, and console.log them. This way I give my fingers, brains, and eyes some practice in seeing using, and using what I’m learning. Here is the link to download, if you don’t have it already: click here (it’s free)
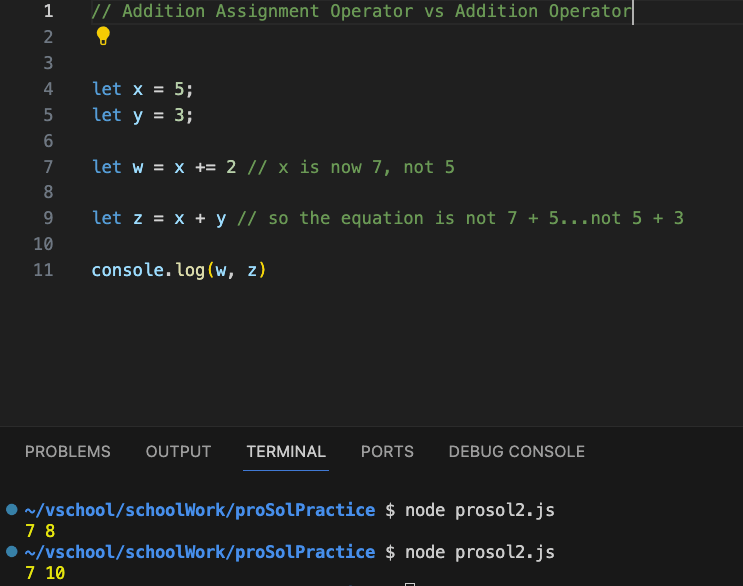

Let’s Practice!
Addition Assignment Operator (+=
):
Addition Operator (+
):
These examples should help clarify how +=
and +
are used in different scenarios within JavaScript.
Well, I hope this was helpful!! I’ve been using and seeing these JavaScript Operators more and more in my coding journey.
Here is additional information from the GOAT W3schools.com!
In summary the Addition Assignment JavaScript Operator ( +=
) is used for updating the value of a variable by adding another value to it, while Addition JavaScript Operator (+
) is used for performing addition or string concatenation, depending on the context in which it’s used.
If you want, make sure to bookmark this page or website for quick references as I’ll be sharing more posts like this!
Hey, fellow code explorers! Your breakdown of += and + operators in JavaScript is spot-on. The practical exercises and hands-on approach with Visual Studio Code add a valuable touch. The bonus tasks involving user input are a great way to apply these concepts. Keep up the excellent work! Looking forward to more posts!
Thanks for checking out my post! Yay, I’m glad you liked it. I’m hoping to make more posts like this to help with hands-on practice for myself and other code explorers☺️.
Hey, fellow code explorers! Your breakdown of += and + operators in JavaScript is spot-on. The practical exercises and hands-on approach with Visual Studio Code add a valuable touch. The bonus tasks involving user input are a great way to apply these concepts. Keep up the excellent work! Looking forward to more posts!
Hi, I just wanted to let you know that I found this to be very helpful. I had been looking at endless YouTube videos on thia, and it’s so time consuming. But this explained what I needed way better. when it comes to coding it can be a giant headache, and super hard and annoying. But this was great and just what I needed. I found it easy to use. Thanks.
I totally understand! YouTube has so much great information, but it’s great to find quick and concise resources as well, especially ones we can practice.
Short and sweet. Thank you for this insightful article.
I took two programming courses as part of my Bachelor’s of Science in Electrical Engineering, but the language we used was C++.
However,I I believe the main operators are pretty much the same across all programming languages, so reading this article unlocked some memories and was a nice refresher.
Thanks for checking it out and I’m glad it unlocked some memories! I’m hoping to keep creating short refreshers like this.
I found your breakdown of additional JavaScript operators really informative! The way you explained the lesser-known operators like the logical nullish assignment (??=) and optional chaining (?.) was clear and easy to follow. Have you encountered situations where these operators significantly improved your coding efficiency? Personally, I’ve found optional chaining incredibly useful when working with nested objects or handling potential null values more gracefully.
Your inclusion of examples and their practical applications was very helpful for understanding the use cases of these operators. How do you prioritize which lesser-known operators to learn and implement in your coding practice? Your insights could guide others in streamlining their learning process. Overall, your post provides a great resource for developers looking to expand their JavaScript knowledge beyond the basics. Great job!
I have so much more to learn as I didn’t even know about (??=) and optional chaining (?.)!! It’s a little intimidating ALL that I don’t know, but also very exciting because I have so much to learn. Thank you for sharing and leaving a comment. Hopefully, my journey will inspire others to start learning tech and coding!